最近在做一个编译原理lab
☝️🤓诶,我已经有结构化的AST和IR了,岂不是能不太费劲地地写出一些有意思的东西?
就这样一拍脑袋给编译器加了个brainfuck后端
基础设施
基本运算符
分支与跳转
文章作者: Cauphenuny
版权声明: 本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来源 Cauphenuny's Blog!
相关推荐
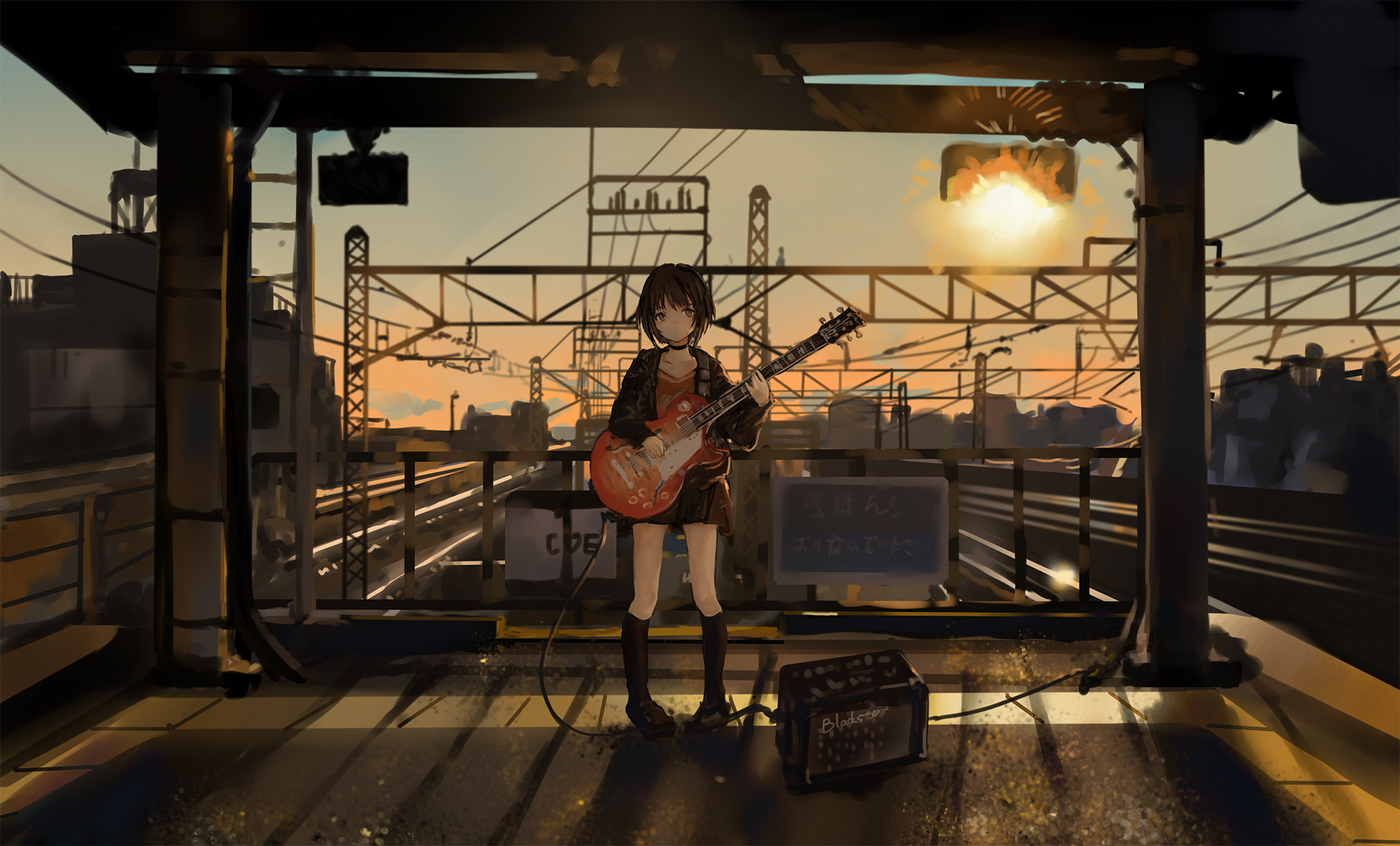
2024-04-19
C++20 新特性试玩:concept
定义 concept 12template<typename T>concept c = (bool_expression); requires(args) {...} 可以作为一个 bool 表达式,检查大括号中内容是否编译通过 e.g. 12template <typename T>concept printable = requires(std::ostream& os, T a) { os << a; }; requires 字句也能嵌套,这样就不用把两个无关的条件参数写到同一个括号里面。 12template <typename T>concept Field = <concepts> 头文件内有一些预定义的...
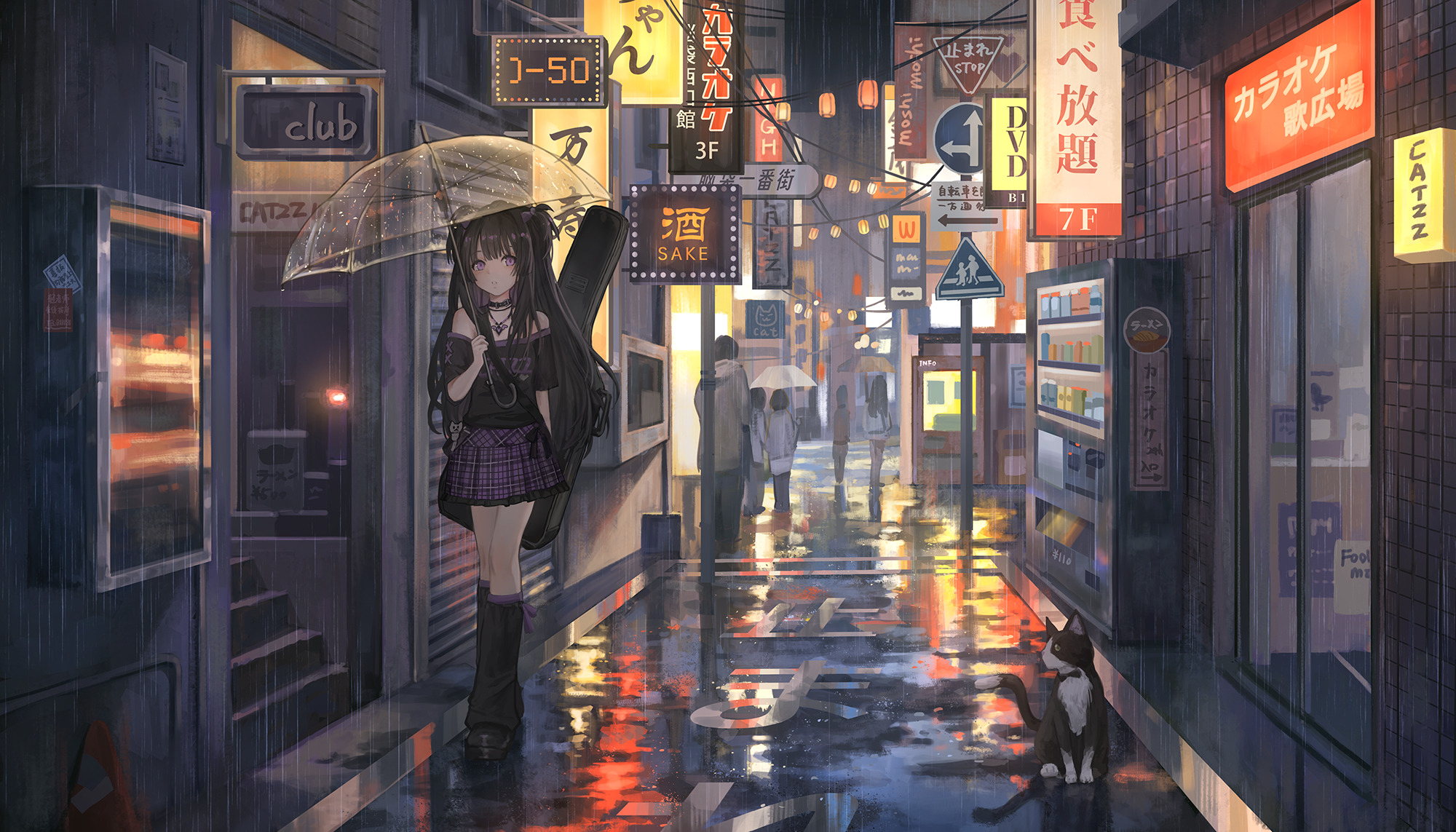
2024-11-08
给C++实现一个模式匹配
没用的小玩具
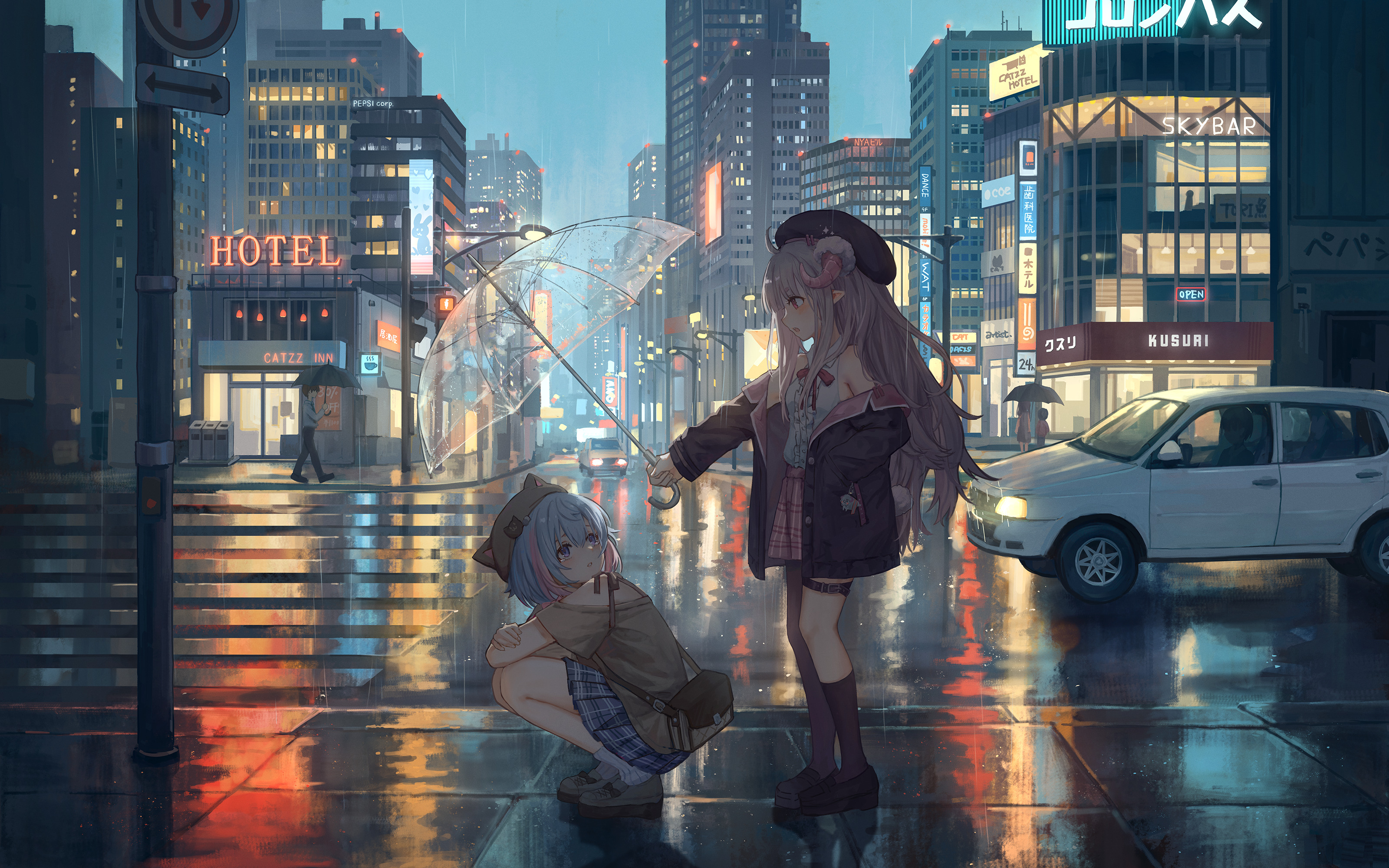
2024-06-14
从零开始打造一个原琴模拟器
计科导大作业 试玩链接: 原琴模拟器 6.14 音色库来源:@Colalala_冰阔落落落 试了半天,发现没法正常播放这个风物之诗琴的音源,决定先用 smplr 默认的钢琴音源把别的写了。 写好了键盘演奏函数、谱子 parser 以及自动播放功能。 version 0.1.0 6.15 加入 [] {} 表示时值变化。 添加临时升降记号 -/+ 以及临时高/低八度记号。 重写了教程 version 0.3.0 6.16 添加了固定调转调方式,更适合对着五线谱演奏。 version 0.4.0 6.17 优化了调号显示。 version 0.4.4 加了个键盘,可以看到哪些音被按下了。 version 0.5.4 把自动演奏和按键动画连接了起来。 version 0.6.0 加了个加载时的提示悬浮窗 version 0.6.1 6.18 重构了代码 version 0.7.0 给按钮加了点阴影,感谢Box-Shadow CSS Generator以及用filter: drop-shadow()给透明图片添加阴影 version 0.7.1 遇到了个...
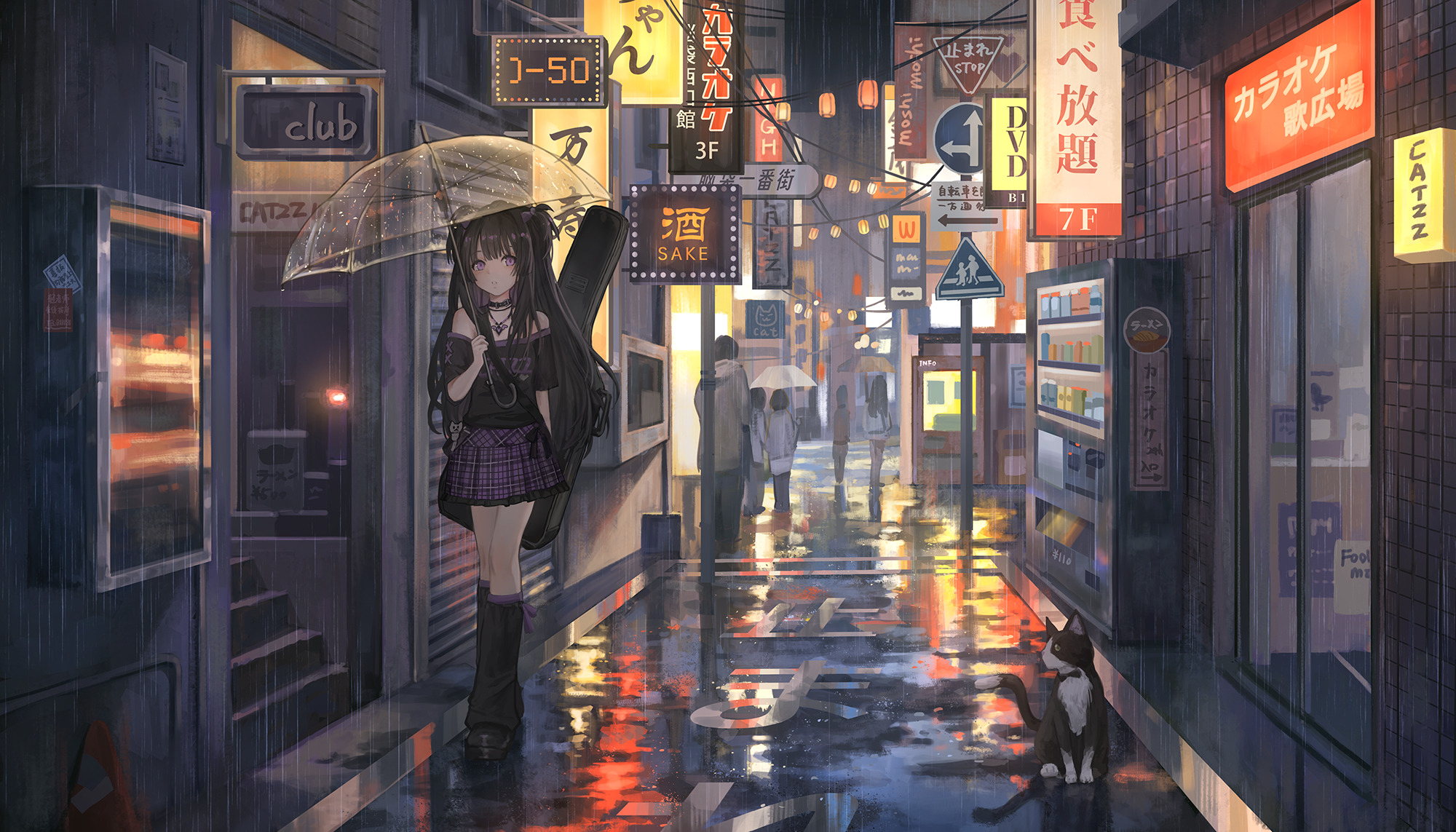
2024-07-05
Xcode CLT 一个有意思的机制
最近觉得终端里面输 python 出来的竟然是 python2 很不爽,然后就想用个软链接把 /usr/local/bin/python 指到 /usr/local/bin/python3。 1$ sudo ln -s /usr/local/bin/python3 /usr/local/bin/python 完事,然后: 好怪哦 弄了半天不知道怎么回事,最后群里问了问,有大佬解释是这个 Xcode CommandLine Tools 提供的 python 会根据传入的程序名称决定行为,argv[0] 是 python 就当 python2 执行 软链接没问题,文件确实是一样的 换个名字就执行不了了 其实我应该自己早点发现这个问题的,之前刷知乎刷到过 clang/clang++ 也是一样的原理,共用一个执行文件,根据传入的 argv[0] 决定是否链接 libc++ 库等 还是没想到这里去 最后用了个 alias ,不折腾软链接了 最后还是用上了 conda 管理环境,删掉了 /usr/local/bin 里面的python/pip等。 等等…… 啊? 所以...
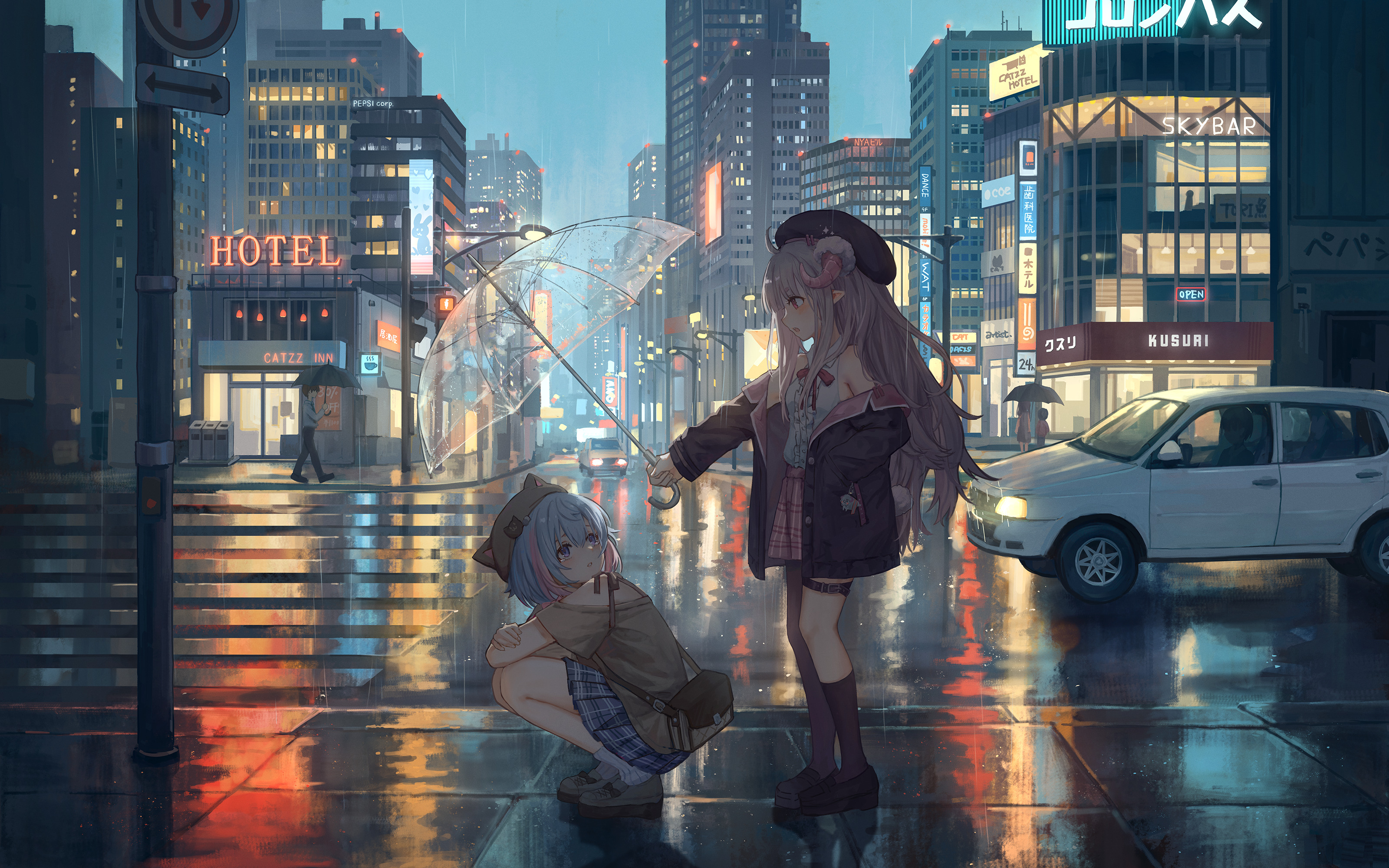
2024-04-12
图灵加法器
题目:loj6572, loj6573, loj6574 主要写一下 t3 一开始本人的思路是先把一个数前后颠倒地放到等号后面,再加上另一个数,最后把求得的和正过来,写了约 90 行。 小组讨论一会后发现这个取反操作挺没必要的,直接把第二个数加到第一个数上就行。 如何处理进位问题? 每次从第二个数那里取一位加至第一个数的时候将一个 0/1 转换为 a/b,相当于打上 “计算完毕”...
评论